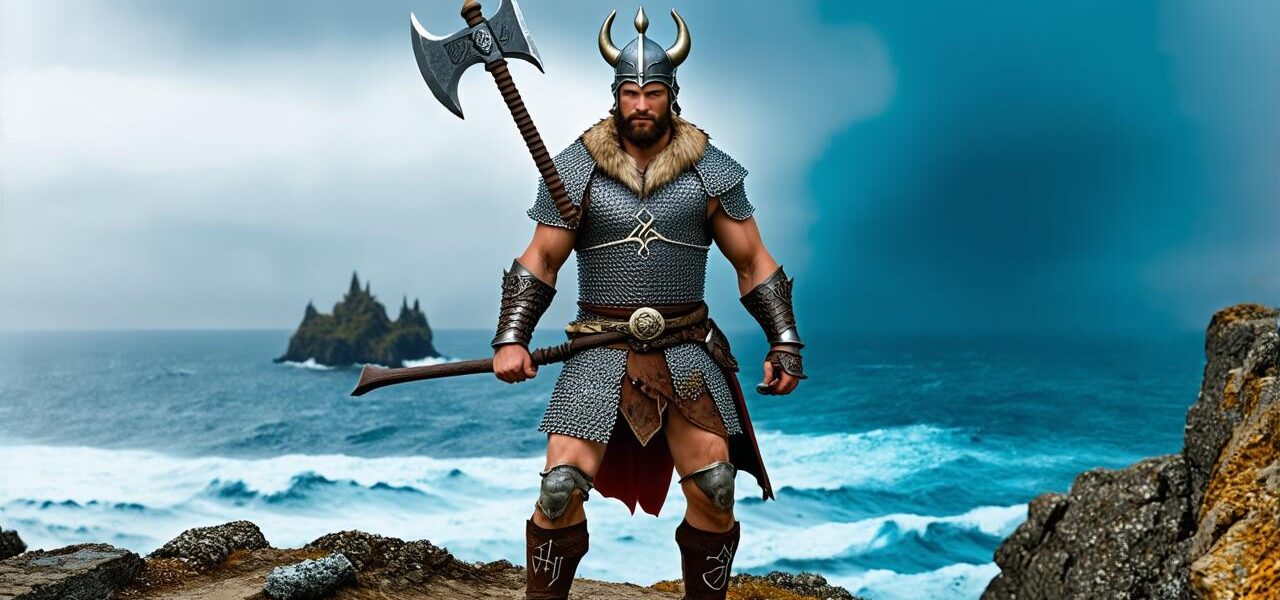
Comprehensive Guide to Blockchain Development: A Step-by-Step Tutorial
Corrected HTML code:
Blockchain technology is revolutionizing various industries around the world, including finance, healthcare, and supply chain management. If you are interested in developing blockchain applications, you need to have a solid understanding of the underlying concepts and technologies. This comprehensive guide will provide you with a step-by-step tutorial on how to develop blockchain applications using Solidity, Ethereum’s smart contract language.
What is Blockchain Development?
Blockchain development involves creating and deploying decentralized applications (dApps) on a blockchain network. These dApps can be used for various purposes, such as storing data, transferring assets, and executing complex business logic. The key feature of blockchain technology is that it allows for secure and transparent transactions without the need for intermediaries.
Why Learn Blockchain Development?
Learning blockchain development can open up new career opportunities in various industries. It can also help you create innovative solutions to complex problems. For example, you could use blockchain technology to develop a secure and transparent voting system or to track the supply chain of a product from manufacturer to end-user. The possibilities are endless!
Prerequisites for Blockchain Development
Before diving into blockchain development, you need to have a basic understanding of programming concepts and data structures. You should also be familiar with JavaScript and have some experience with web development. Additionally, it would be helpful to have some knowledge of cryptography and distributed systems.
Getting Started with Solidity and Ethereum
Solidity is a smart contract language that runs on the Ethereum blockchain. Ethereum is a decentralized platform that allows developers to build dApps without relying on intermediaries. To get started with Solidity and Ethereum, you need to install the Ethereum client software, such as Ganache or Truffle. Once you have installed the client software, you can create a new account and start writing smart contracts in Solidity.
Understanding Smart Contracts in Solidity
A smart contract is a self-executing program that runs on the blockchain. It consists of a set of rules that define how data is stored and processed on the blockchain. In Solidity, you can use various keywords to define the structure and logic of your smart contracts. For example, you can use the "contract" keyword to define a new contract, the "function" keyword to define a function that can be called by other contracts or dApps, and the "modifier" keyword to add additional security features to your contract.
Creating a Simple Smart Contract in Solidity
Let’s create a simple smart contract in Solidity that allows users to transfer Ether (ETH) between two addresses on the Ethereum blockchain.
solidity
pragma solidity ^0.8.0;
contract TransferEther {
mapping(address => uint256) public balances;
function transfer(address payable to, uint256 amount) external {
require(msg.value > amount, "Insufficient funds");
balances[to] += amount;
balances[msg.sender] -= amount;
}
}
In this contract, we define a mapping called "balances" that stores the Ether balance of each address on the blockchain. We also define a function called "transfer" that allows users to send Ether from one address to another. The function checks whether the sender has sufficient funds and updates the balances accordingly.
Deploying the Smart Contract on Ethereum
To deploy the smart contract on Ethereum, you need to compile it using a Solidity compiler and then use the client software to send the compiled bytecode to the Ethereum network. Once the bytecode is executed on the blockchain, the contract becomes accessible to other users and can be used to transfer Ether between addresses.
Building a Decentralized Application (dApp) using Solidity
Now that you have created your first smart contract, let’s build a dApp using it. The dApp will allow users to send Ether to each other using the "transferEther" function we defined earlier.
solidity
pragma solidity ^0.8.0;
contract TransferEther {
mapping(address => uint256) public balances;
function transfer(address payable to, uint256 amount) external {
require(msg.value > amount, "Insufficient funds");
balances[to] += amount;
balances[msg.sender] -= amount;
}
}
contract TransferEtherApp {
mapping(address => TransferEther) public transferEthers;
function send(address to, uint256 amount) external {
require(transferEthers[msg.sender].transfer(to, amount), "Transfer failed");
}
}
In this dApp, we define a new contract called "TransferEtherApp" that wraps around the "TransferEther" contract we defined earlier. The "TransferEtherApp" contract provides an interface for users to send Ether to each other using the "send" function. When a user calls the "send" function, it checks whether the transfer was successful and returns an error message if it wasn’t.
Interacting with the dApp using Ethereum Wallets
To interact with the dApp, you need to have an Ethereum wallet that contains Ether. There are many different wallets available, including MetaMask and MyEtherWallet. Once you have set up a wallet, you can use it to call the "send" function on the dApp and transfer Ether between addresses.
Conclusion
Blockchain development is a rapidly growing field with countless opportunities for innovation and entrepreneurship. By learning Solidity and Ethereum