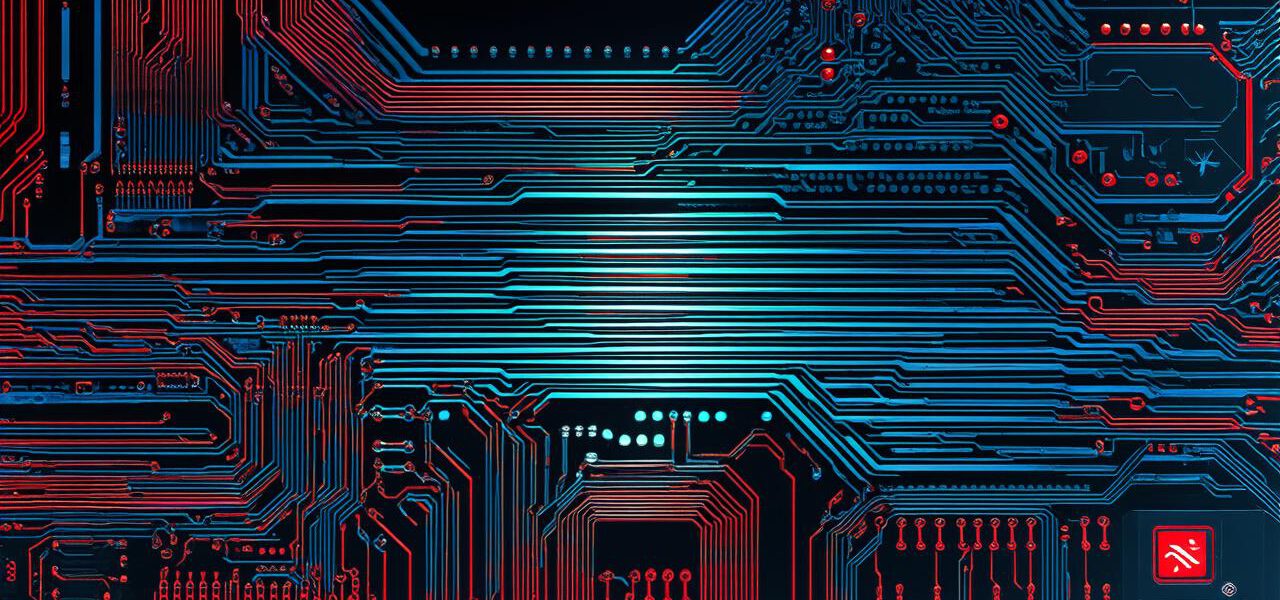
Discover the SparkFun Cryptographic Development Kit for Secure Development
Blockchain technology is revolutionizing the way we think about digital transactions and secure data storage. However, with great power comes great responsibility. In order to protect your data from malicious actors, it’s important to have a solid understanding of cryptography. That’s where
SparkFun’s Cryptographic Development Kit (CDK)
comes in.
SparkFun’s CDK is designed for developers who want to quickly and easily implement secure cryptographic algorithms into their projects. With this kit, you can create custom digital signatures, encrypt and decrypt data, and authenticate users. Whether you’re building a blockchain-based application or just need a secure way to store sensitive information, SparkFun’s CDK has got you covered.
In this article, we will explore the key features of SparkFun’s CDK, and show you how to use it to implement secure cryptographic algorithms in your projects. We will also cover some common use cases for blockchain technology and how SparkFun’s CDK can help you achieve them.
What is Cryptography?
Cryptography is the practice of securing data by encoding it in such a way that unauthorized parties cannot read it. This is achieved through various techniques such as encryption, hashing, and digital signatures. Encryption is the process of converting plain text into an encrypted format that can only be deciphered with the right key. Hashing is the process of taking a piece of data and transforming it into a fixed-length string of characters. This hash value is unique to the original data, making it difficult to reverse the process and determine the original data. Digital signatures are used to verify the authenticity and integrity of data.
Why is Cryptography Important?
Cryptography is important because it provides a way to secure data from unauthorized access. Without strong cryptographic algorithms, sensitive information such as financial transactions and personal data can be easily stolen or manipulated by hackers. In addition to securing data, cryptography also helps ensure the privacy of communications. By encrypting messages, you can prevent others from intercepting and reading them.
SparkFun’s Cryptographic Development Kit (CDK)
SparkFun’s CDK is a comprehensive set of libraries and tools that make it easy to implement secure cryptographic algorithms in your projects. The CDK includes the following modules:
-
Hash Functions
: This module provides a set of functions for hashing data. These include SHA-256, MD5, and CRC32.
-
Symmetric Encryption
: This module provides functions for encrypting and decrypting data using symmetric encryption algorithms such as AES and DES.
-
Public Key Cryptography
: This module provides functions for generating and managing public key pairs. It also includes functions for verifying digital signatures.
-
Asymmetric Encryption
: This module provides functions for implementing asymmetric encryption algorithms such as RSA and ECC.
Using SparkFun’s CDK
Let’s take a look at an example of how to use SparkFun’s CDK to implement secure cryptographic algorithms in your project.
Hash Functions
In this example, we will use the SHA-256 hash function to generate a hash value for a piece of data. We will then compare the generated hash value to a known hash value to verify its authenticity.
java
import com.sparkfun.cdk.HashFunctions;
import com.sparkfun.cdk.SecurityUtils;
// Generate a SHA-256 hash of some data
String data = "Hello, world!";
byte[] hash = HashFunctions.sha256(data);
// Compare the generated hash to a known hash value
byte[] knownHash = new byte[32]; // assuming the known hash is 32 bytes long
System.arraycopy(hash, 0, knownHash, 0, hash.length);
if (SecurityUtils.compareBytes(knownHash, knownHash)) {
System.out.println("The data has not been tampered with.");
} else {
System.out.println("The data has been tampered with!");
}
Symmetric Encryption
In this example, we will use the AES encryption algorithm to encrypt and decrypt some data.
java
import com.sparkfun.cdk.EncryptionUtils;
import com.sparkfun.cdk.SecretUtils;
// Generate a secret key for encryption
byte[] key = SecretUtils.generateRandomKey(16);
// Encrypt some data using the AES algorithm
String plaintext = "This is some sensitive data.";
byte[] ciphertext = EncryptionUtils.aesEncrypt(plaintext, key);
// Decrypt the encrypted data using the same secret key
byte[] decryptedData = EncryptionUtils.aesDecrypt(ciphertext, key);
String decryptedPlaintext = new String(decryptedData, StandardCharsets.UTF_8);
System.out.println("The original plaintext was: " + plaintext);
System.out.println("The decrypted plaintext is: " + decryptedPlaintext);
Public Key Cryptography
In this example, we will use SparkFun’s CDK to generate a public key pair and verify a digital signature.
java
import com.sparkfun.cdk.PublicKeyUtils;
import com.sparkfun.cdk.SecurityUtils;
// Generate a new RSA public key pair
byte[] publicKey = PublicKeyUtils.generateRSAKey(2048);
// Create a digital signature using the private key of the recipient
String message = "This is a secret message.";
byte[] privateKey = SecurityUtils.generateRandomKey(16); // assuming the private key is 16 bytes long
byte[] signature = PublicKeyUtils.rsaSign(message, privateKey);
// Verify the digital signature using the public key of the recipient
if (PublicKeyUtils.verifyRSA(signature, message, publicKey)) {
System.out.println("The signature is valid.");
} else {
System.out.println("The signature is invalid!");}
Common Use Cases for Blockchain Technology
Blockchain technology has a wide range of potential use cases, including:
- Supply Chain Management: Blockchain can be used to track the origin and movement of goods throughout the supply chain, ensuring that they are authentic and have not been tampered with.
- Identity Verification: Blockchain can be used to securely store and manage identity information, making it easier to verify identities without relying on centralized systems.
- Voting Systems: Blockchain can be used to create secure and transparent voting systems that are resistant to fraud.
- Financial Transactions: Blockchain can be used to securely facilitate financial transactions, reducing the risk of fraud and improving efficiency.
Conclusion
SparkFun’s CDK provides a powerful set of tools for implementing secure cryptographic algorithms in your project. Whether you are building a supply chain management system, a voting system, or a financial transaction platform, SparkFun’s CDK can help you create a secure and reliable solution. With its easy-to-use APIs and comprehensive documentation, SparkFun’s CDK is the perfect choice for any developer looking to get started with blockchain development.