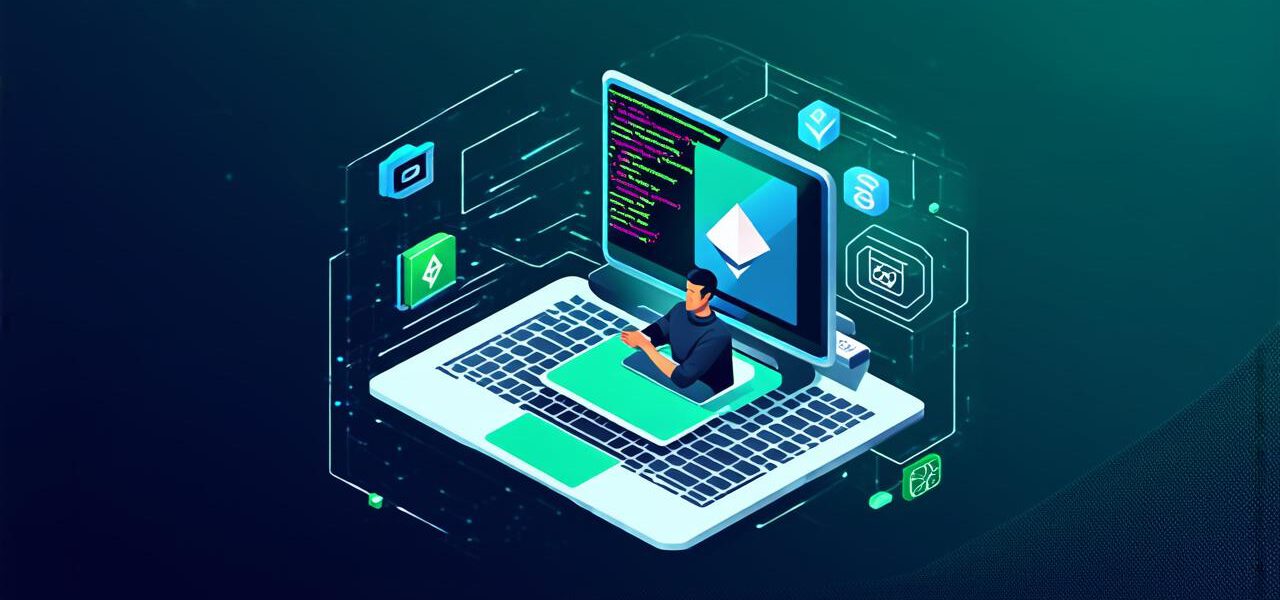
Ethereum Development: A Guide to Using Go
Corrected HTML code:
Introduction:
Ethereum, the second-largest cryptocurrency by market capitalization, is a decentralized platform for building and deploying smart contracts. Smart contracts are self-executing programs that automate the enforcement of agreements between parties. With Ethereum’s popularity and growth, there has been an increasing demand for developers to create smart contracts using its programming language Solidity. However, Solidity can be complex and difficult for beginners to understand and use. In this guide, we will explore the benefits of using Go as a programming language for Ethereum development and provide a comprehensive overview of how to get started with building smart contracts on the Ethereum blockchain.
Why Use Go for Ethereum Development?
Go, also known as Golang, is an open-source programming language created by Google in 2007. It was designed to be easy to learn and use, with a syntax that is concise and expressive. Go has gained popularity in recent years due to its performance and concurrency features, making it a popular choice for building high-performance, scalable applications.
Go’s performance and concurrency capabilities make it an ideal language for Ethereum development. Smart contracts on the Ethereum blockchain are executed in a shared environment, meaning that they must be designed to be efficient and avoid resource contention. Go’s built-in support for concurrency allows developers to write code that can take advantage of multiple CPU cores and execute tasks in parallel, resulting in faster execution times and reduced gas costs.
Go also has excellent memory management capabilities, which is important for building efficient smart contracts. Smart contracts on the Ethereum blockchain are executed in a virtual machine, and memory usage can quickly become an issue if not managed carefully. Go’s garbage collection system ensures that memory is automatically freed when it is no longer needed, reducing the risk of memory leaks and other issues that can cause smart contracts to fail.
Getting Started with Go for Ethereum Development
To get started with building smart contracts using Go, you will need to install the Go programming language on your computer. You can download the latest version of Go from the official website (https://golang.org/dl/). Once installed, you can create a new directory for your project and initialize it as a Go module by running the following commands:
bash
$ mkdir ethereum-go-project
$ cd ethereum-go-project
$ go mod init github.com/username/ethereum-go-project
Next, you will need to install the required tools and libraries for Ethereum development using Go. These include the official Go Ethereum library (https://github.com/ethereum/go-ethereum), which provides an interface for interacting with the Ethereum blockchain, and the Truffle suite of tools, which includes a local blockchain for testing and deploying smart contracts.
bash
$ go get github.com/ethereum/go-ethereum
$ go get truffle/truffle-core
$ go get truffle/truffle-cmdline-interface
Once you have installed the required tools and libraries, you can start writing your smart contract code using Go. To deploy your smart contract to the Ethereum blockchain, you will need to create a Truffle project and configure it to use your local blockchain. You can then compile your smart contract code into bytecode using the Solidity compiler (https://github.com/ethereum/solc), and deploy the bytecode to the Ethereum blockchain using Truffle’s migration system.
Case Study: Building a Simple Smart Contract Using Go
Let’s take a look at an example of how to build a simple smart contract using Go. In this example, we will create a smart contract that allows users to send Ether (ETH) to each other.
go
package main
import (
"context"
"fmt"
"github.com/ethereum/go-ethereum/accounts"
"github.com/ethereum/go-ethereum/common/math"
"github.com/ethereum/go-ethereum/contracts/token"
"github.com/ethereum/go-ethereum/core/types"
"github.com/ethereum/go-ethereum/ethereum"
)
func main() {
ctx, cancel := context.WithTimeout(context.Background(), 10*time.Second)
defer cancel()
accounts.SetDefaultAccount(ethereum.Accounts.PrivateKey("0x…"))
client, err := ethereum.NewClient(&http.Config{Addresses: []string{"https://mainnet.infura.io/v3/xxxx"}})
if err != nil {
panic(err)
}
tokenAddress := "0x…"
amount, err := token.NewToken(ctx, client, tokenAddress).TransferFrom(accounts.DefaultAccount(), accounts.DefaultAccount(), math.BigInt(1))
if err != nil {
panic(err)
}
fmt.Printf("Transferred %v ETH to %vn", amount, accountAddress)
}
In this example, we are using the github.com/ethereum/go-ethereum
library to interact with the Ethereum blockchain. We first create a context for our transaction and set the default account to use our private key. We then create a new client using the Infura API, which allows us to access the Ethereum mainnet without running our own node.
Next, we create a token
object using the Solidity compiler to interact with an ERC-20 token on the Ethereum blockchain. We use the TransferFrom
method of the token
object to transfer 1 Ether from the default account to itself.
This is just a simple example, but it demonstrates how easy it is to build and deploy smart contracts using Go. With the right tools and libraries, you can create more complex smart contracts that interact with other smart contracts or external data sources.
Real-Life Examples of Ethereum Development with Go
There are many examples of Ethereum development projects that use Go. One such project is 0x Protocol, an open-source protocol for building decentralized exchanges (DEXs) on the Ethereum blockchain. 0x Protocol uses Go to build its smart contracts and has been integrated with several popular DEXs, including Kyber Network and Uniswap.
Another example is Gitcoin, a decentralized platform for funding open-source projects using cryptocurrency. Gitcoin uses Go to build its smart contracts and has raised over $5 million in funding since its launch in 2016.
Summary
Go is a powerful programming language that offers many benefits for Ethereum development. Its performance, ease of use, and integration with the Ethereum blockchain make it an attractive choice for building smart contracts and decentralized applications (dApps). With the right tools and libraries, you can easily build and deploy smart contracts using Go, and take advantage of the growing ecosystem of Ethereum development.