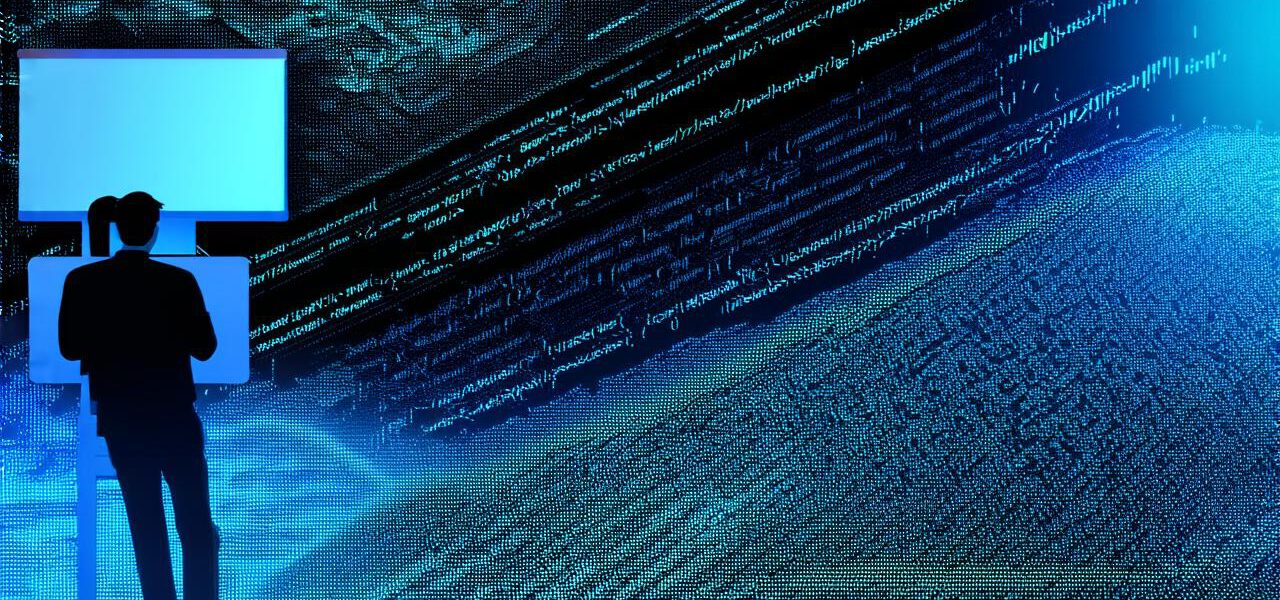
How can Python be used for blockchain development?
Blockchain technology is quickly transforming the way we store and share data, making it possible to create secure, transparent, and decentralized systems. However, building a blockchain requires specialized skills and knowledge, which can be hard to come by. That’s where Python comes in.
Python is a popular programming language that’s used for a wide range of applications, including web development, data science, and machine learning. It’s also gaining popularity in the blockchain community due to its simplicity and ease of use. In this guide, we’ll explore how Python can be used for blockchain development, from building smart contracts to creating decentralized applications (dApps).
Getting Started with Python for Blockchain Development
Before you dive into the world of blockchain development using Python, you need to have a basic understanding of the language. Here are some resources that can help you get started:
- Python tutorial
- Python for data science
Once you have a good grasp of the basics, it’s time to start learning about the specific libraries and frameworks that are used for blockchain development. Here are some popular options:
- PyCoin – A Python library for creating simple blockchains.
- Web3.py – A Python library for interacting with the Ethereum blockchain.
- EthereumJS – A JavaScript library for building Ethereum-based applications that can be used with Python through a bridge like PyEthJS.
Building Smart Contracts with Python
Smart contracts are self-executing programs that run on the blockchain and can be used to automate complex processes. They are written in a programming language that’s specific to the blockchain platform, such as Solidity for Ethereum or Vyper for Hyperledger Fabric. However, Python can also be used to create smart contracts, thanks to libraries like PyEthJS and Truffle Suite.
Here’s an example of a simple smart contract written in Python using PyCoin:
python
from pycoin import Blockchain
Create a new blockchain
blockchain = Blockchain()
Define the smart contract
def my_contract():
Do something here
pass
Call the smart contract function
my_contract()
Building Decentralized Applications (dApps) with Python
dApps are applications that run on the blockchain and can be accessed through a web browser or a mobile app. They can be used for a wide range of purposes, such as buying and selling goods, exchanging cryptocurrencies, and voting.
Python can be used to build dApps using frameworks like EthereumJS and Truffle Suite. Here’s an example of how you might use EthereumJS to create a simple dApp:
python
from ethers import Account, HDWalletProvider
from web3.eth.abi import ABCI
Define the ABI (Application Binary Interface) for the dApp
ABCI = [
{
"constant": false,
"inputs": [],
"name": "balanceOf",
"outputs": ["uint256"],
"payable": false,
"stateMutability": "view",
"type": "function"
}
]
Create a new account and HDWalletProvider for the dApp
account = Account.from_private_key("0x…"")
provider = HDWalletProvider(account)
Connect to the Ethereum network
web3 = Web3(provider)
Define the dApp contract
contract = web3.eth.contract(address="0x…", ABI=ABCI)
Call the dApp contract function
balance = contract.functions.balanceOf().call({"from": account.address})
print("Your balance:", balance)
Case Studies of Python in Blockchain Development
Here are some real-life examples of how Python has been used for blockchain development:
- Coinbase – Coinbase, one of the largest cryptocurrency exchanges in the world, uses Python extensively for its backend and trading platforms.
- Chainlink – Chainlink is a decentralized oracle network that provides data feeds to smart contracts on various blockchains. It was built using Python and other programming languages.
- Bitcoin Magazine – Bitcoin Magazine, a leading publication covering the world of bitcoin and blockchain technology, uses Python for its web development and data analysis needs.
FAQs
Here are some frequently asked questions about using Python for blockchain development:
- What kind of projects can be built using Python for blockchain development?
- Smart contracts
- dApps
- Decentralized finance (DeFi) applications
- Supply chain management systems
- Predictive analytics and data analysis
- What libraries and frameworks are best suited for Python in blockchain development?