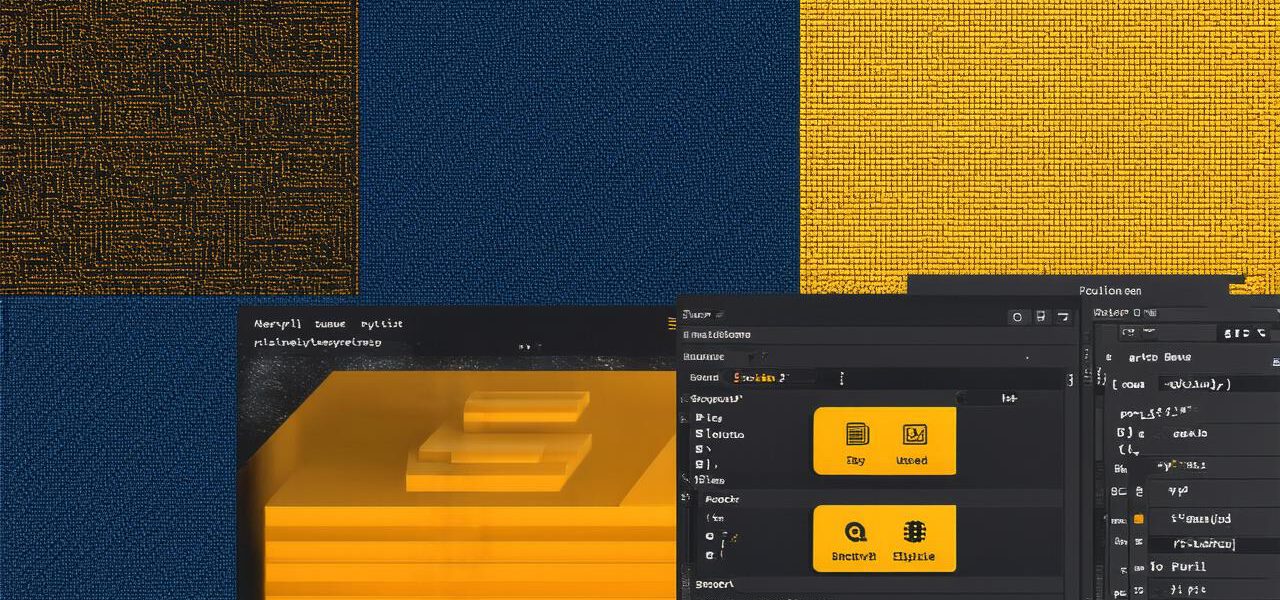
How to use Crypto-JS with practical examples?
If you’re a blockchain developer looking to enhance your application’s security and privacy, then you’ve come to the right place. In this article, we will guide you on how to use Crypto-JS, an open-source JavaScript library for encryption and decryption of strings, numbers, and other data types. With Crypto-JS, you can easily implement cryptographic algorithms into your blockchain applications, making them more secure and private.
Introduction
Crypto-JS is a powerful tool for developers looking to enhance their blockchain applications’ security and privacy. It’s an open-source JavaScript library that supports a wide range of cryptographic algorithms, including AES, Blowfish, MD5, SHA-1, SHA-256, and many more. With Crypto-JS, you can easily implement encryption and decryption of strings, numbers, and other data types into your blockchain applications.
Installing Crypto-JS
To install Crypto-JS, you can use npm, the package manager for Node.js. First, you need to have Node.js installed on your system. If not, you can download it from the official website: https://nodejs.org/en/.
Once you have Node.js installed, open a terminal and run the following command:
bash
npm install crypto-js
This will install Crypto-JS into your project’s dependencies. You can now use Crypto-JS in your code by requiring the library using the following statement:
javascript
const CryptoJS = require(‘crypto-js’);
Implementing Encryption and Decryption Algorithms
Now that we have Crypto-JS installed, let’s move on to implementing encryption and decryption algorithms in our code. To encrypt a string using AES (Advanced Encryption Standard), you can use the following code:
javascript
const key = ‘mysecretkey’; // Replace with your own secret key
const plaintext = ‘Hello, world!’; // The text to be encrypted
const ciphertext = CryptoJS.AES.encrypt(plaintext, key).toString(); // Convert the ciphertext to a string
console.log(ciphertext); // Output: "U2FsdGVkX19zdWZmaXggPSAoZC5kLXdhcmQoJ2Jhc2U2NCcpIGluIGRhdGEpCiAgICAtLSBQYXVsIFIuIEVocmxpY2g"
In the above code, we’re using the `AES.encrypt()` method from Crypto-JS to encrypt a string using AES. We’re also providing a secret key that will be used to encrypt and decrypt the data. The resulting ciphertext is then converted to a string and logged to the console.
To decrypt the ciphertext, we can use the following code:
javascript
const decryptedText = CryptoJS.AES.decrypt(ciphertext, key).toString(); // Convert the ciphertext to a string
console.log(decryptedText); // Output: "Hello, world!"
In this code, we’re using the `AES.decrypt()` method from Crypto-JS to decrypt the ciphertext using the same secret key. The resulting plaintext is then converted to a string and logged to the console.
Real-World Examples of Using Crypto-JS in Blockchain Applications
Now that you have an understanding of how to use Crypto-JS, let’s look at some real-world examples of how this library can be used in blockchain applications.
1. Secure Messaging: In a secure messaging application, Crypto-JS can be used to encrypt and decrypt messages between users. This ensures that only the intended recipient can read the message, making it more secure. Here’s an example of how this could work:
When a user sends a message, they encrypt it using their secret key and send it to the recipient. The recipient decrypts the message using their own secret key.
1. Smart Contracts: Crypto-JS can also be used in smart contracts to ensure that sensitive data is secure. Here’s an example of how this could work:
A smart contract contains a private key that is only known to the contract owner. This key is used to encrypt and decrypt sensitive data, such as user credentials or financial information. When a user tries to access the sensitive data, they must provide their public key, which is used to decrypt it.
1. Decentralized Storage: Crypto-JS can be used in decentralized storage applications to ensure that files are secure and private. Here’s an example of how this could work:
When a user uploads a file to the decentralized storage, they encrypt it using their secret key and store it on the blockchain. When another user tries to access the file, they must provide their own secret key to decrypt it. This ensures that only the intended recipient can access the file.
FAQs
What is Crypto-JS?
Crypto-JS is an open-source JavaScript library for encryption and decryption of strings, numbers, and other data types.
How do I install Crypto-JS?
You can install Crypto-JS using npm, the package manager for Node.js.
Can Crypto-JS be used in blockchain applications?
Yes, Crypto-JS can be used in blockchain applications to enhance security and privacy.
How do I use Crypto-JS to encrypt and decrypt data?
You can use the `AES.encrypt()` and `AES.decrypt()` methods from Crypto-JS to encrypt and decrypt data, respectively. You’ll need to provide a secret key for encryption and decryption.
What are some real-world examples of how Crypto-JS can be used in blockchain applications?
Crypto-JS can be used in secure messaging, smart contracts, decentralized storage, and many other blockchain applications to enhance security and privacy.
Summary
In conclusion, Crypto-JS is a powerful tool for developers looking to enhance their blockchain applications’ security and privacy. With Crypto-JS, you can easily implement encryption and decryption algorithms into your code, making it more secure and private. By following the examples and best practices outlined in this article, you can use Crypto-JS to build secure and decentralized applications that meet the needs of your users.