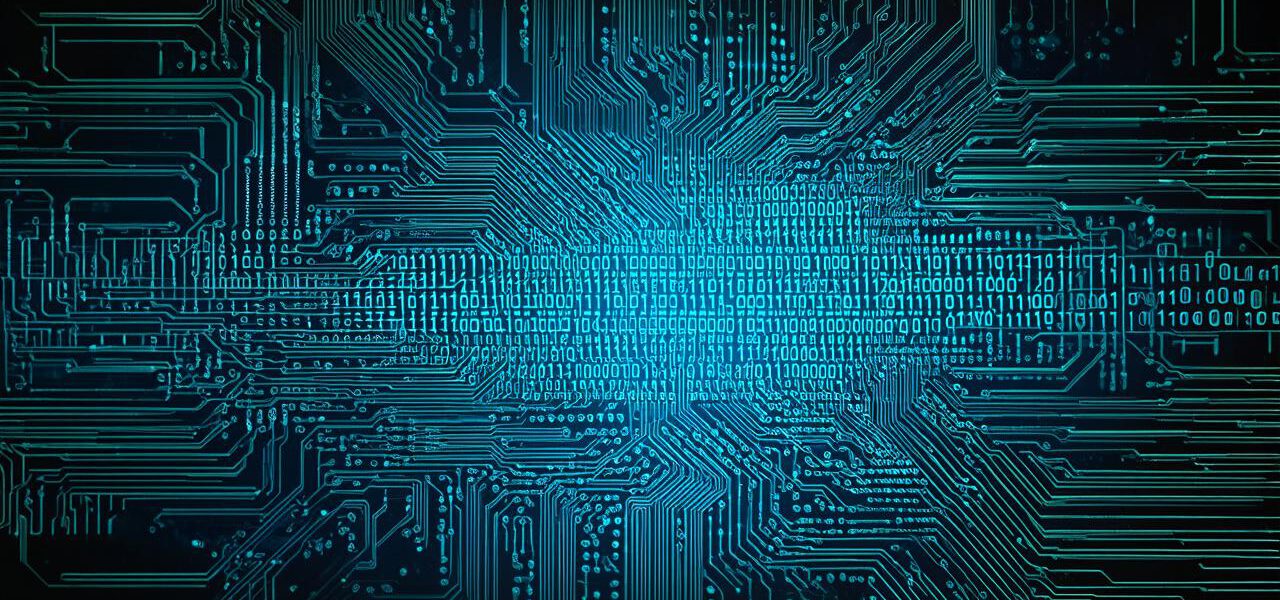
Learn about blockchain development using C++ for advanced programming
Introduction
Blockchain technology is revolutionizing the way we store and share data across various industries. It offers an immutable, decentralized, and secure platform to build applications that enable peer-to-peer transactions without intermediaries.
In this article, we will explore how C++ can be used to develop advanced blockchain applications that offer high performance and scalability. We will discuss the benefits of using C++ for blockchain development, including its low-level control, concurrency support, and memory management capabilities. Additionally, we will provide a detailed guide on how to build a simple blockchain using C++, along with examples and case studies to illustrate the concepts.
Benefits of Using C++ for Blockchain Development
C++ is a high-performance programming language that offers low-level control over memory management, concurrency, and other system resources. These features make it an ideal choice for building blockchain applications that require high throughput and scalability.
Here are some of the benefits of using C++ for blockchain development:
- Low-Level Control: C++ offers developers full control over the underlying hardware and memory management, allowing them to optimize performance and memory usage. This is especially important in blockchain development, where every transaction requires complex computations and memory management.
- Concurrency Support: Blockchain applications require concurrent access to shared data structures and resources. C++ provides built-in support for concurrency through threads and synchronization primitives such as locks, semaphores, and atomic variables. This allows developers to build blockchain applications that can handle multiple transactions simultaneously without compromising on performance or safety.
- Memory Management Capabilities: Blockchain applications require complex data structures that need to be managed efficiently. C++ provides advanced memory management capabilities, including smart pointers and automatic garbage collection, which make it easier for developers to manage large and complex data structures. This is especially important in blockchain development, where every transaction requires access to the entire ledger.
Building a Simple Blockchain using C++
Now that we have discussed the benefits of using C++ for blockchain development, let’s take a look at how to build a simple blockchain using C++. We will use a proof-of-work consensus algorithm to illustrate the concepts.
Proof-of-Work Consensus Algorithm
The proof-of-work consensus algorithm is one of the most commonly used algorithms in blockchain development. It requires miners to compete to solve a complex mathematical problem, which proves that they have spent a certain amount of computational resources on validating transactions. Once a miner solves the problem, they are rewarded with new coins and their solution is added to the blockchain.
To implement a proof-of-work consensus algorithm in C++, we will use the SHA-256 hashing function to compute the nonce for each block. Here’s how the process works:
- Create a genesis block with a fixed nonce value of 0.
- For each subsequent block, calculate the nonce value by iterating through all previous blocks and adding their hash values and timestamps to the current block’s data.
- Calculate the SHA-256 hash of the current block using the nonce value.
- If the SHA-256 hash meets a certain difficulty threshold (i.e., it takes a certain amount of time to compute), then the block is considered valid and added to the blockchain.
Example Implementation
c++
include
include
include
include
include <openssl/sha.h>
struct Block {
int index;
time_t timestamp;
std::string data;
int nonce;
std::string prevHash;
std::string hash;
};
class Blockchain {
public:
Blockchain() {
genesisBlock.index = 0;
genesisBlock.timestamp = time(NULL);
genesisBlock.data = "Genesis block";
genesisBlock.nonce = 0;
genesisBlock.prevHash = "";
genesisBlock.hash = computeHash(genesisBlock);
}
void addBlock(const std::string& data) {
Block newBlock;
newBlock.index = blockchain.size();
newBlock.timestamp = time(NULL);
newBlock.data = data;
newBlock.nonce = 0;
newBlock.prevHash = blockchain[newBlock.index – 1].hash;
newBlock.hash = computeHash(newBlock);
while (newBlock.hash.substr(0, 4) != "0000") {
newBlock.nonce++;
newBlock.hash = computeHash(newBlock);
}
blockchain.push_back(newBlock);
}
private:
std::vector blockchain;
std::string computeHash(const Block& block) const {
std::stringstream ss;
ss << block.index << block.timestamp << block.data << block.nonce << block.prevHash;
return sha256(ss.str());
}
static std::string sha256(const std::string& input) {
unsigned char digest[SHA256_DIGEST_LENGTH];
SHA256_CTX ctx;
SHA256_Init(&ctx);
SHA256_Update(&ctx, input.c_str(), input.size());
SHA256_Final(digest, &ctx);
std::stringstream ss;
for (int i = 0; i < SHA256_DIGEST_LENGTH; ++i) {
ss << std::hex << std::setw(2) << std::setfill(‘0’) << static_cast(digest[i]);
}
return ss.str();
}
Block genesisBlock;
};
int main() {
Blockchain blockchain;
std::cout << "Mining block 1…" << std::endl;
blockchain.addBlock("This is a test block");
std::cout << "Mining block 2…" << std::endl;
blockchain.addBlock("Another test block");
// Print the blockchain
for (const auto& block : blockchain) {
std::cout << "Index: " << block.index << ", Timestamp: " << ctime(&block.timestamp) << ", Data: " << block.data << ", Nonce: " << block.nonce << ", Previous Hash: " << block.prevHash << ", Hash: " << block.hash << std::endl;
}
return 0;
}
This implementation uses the SHA-256 hashing function to compute the nonce value and hash of each block. The addBlock
method checks whether the computed hash meets a difficulty threshold (in this case, the first four characters of the hash must be "0000"). If it does, then the block is considered valid and added to the blockchain; otherwise, the nonce value is incremented and the process repeats until a valid block is found.